Hello all, Library Management system is a simple yet fantastic project which keeps track of each book present in a library as well as the books which are issued, returned by the reader. It is an essential piece of software required by schools and colleges to maintain and library record of students.
📌 Why you should make this project ~ The peoples who want to enhance their python skills then this sort of project are very nice to try and build and the students in college can present this project in their DBMS system.
Table of Contents
We are going to make a complete library Management system project using python and GUI using a popular module named Tkinter which is so interactive in nature and for the database, we will use MySQL for sake of simplicity.
👉 If you do not have MySQL installed then, please download it from here
What is Tkinter?
Python offers a huge st of libraries to design a GUI interface and one such amazing utility is Tkinter. Tkinter is an open-source Python module used for building GUI applications. Developing desktop-based applications using python Tkinter is not a complex task, you can simply create a top-level window and apply your designs. It contains a huge set of widgets like buttons, check buttons, canvas, frame, labels, and many more.
Library Management System in Python
We have to understand the introduction of our project, now let's jump into its implementation part. The final project will look something like this with each CRUD operation running on the system.
Project Prerequisites
- Tkinter: pip install Tkinter
- pillow: pip install pillow
- pymysql: pip install pymysql
To work with pymysql, you need MySQL installed. Instead of pyMySQL, you can also use MySQL.connector library you like that, all the methods are same.
Description of Project files:
Below are the project files you need to make to complete the project. you can also download the complete source code from the link provided at end of the article.
- main.py: file which does function calling to all other functions and it is our home page of the project.
- AddBook.py: a program that performs the task of adding new books to the library.
- ViewBooks.py: To view the complete list of books in a library
- DeleteBook.py: To Delete a book from the library.
- IssueBook.py: To Issue a book from the library.
- ReturnBook.py: To Return a book to a library.
We will implement each and every function step by step and understand which performs what task. so please be calm and enjoy the project. let's dive in.
Create The Database and Tables
First, in your MySql create a new database and create the following tables which will be used to perform database operations. One by one run the below requires creating two new tables in our database.
create database mydb;
use mydb;
create table books(bid varchar(20) primary key, title varchar(30), author varchar(30), status varchar(30));
create table books_issued(bid varchar(20) primary key, issueto varchar(30));
Implement the Library Management, Coding
let's start the detailed discussion of each file and function one by one.
1) main.py (Home Page)
Import Modules
we have installed a 3 module, now to work with them, import them first.
Connect to MySQL server
Designing a Window
Now, we will design a project window add a background image to it and then, add buttons one by one as we have seen in the image.
root = Tk()
root.title("Library")
root.minsize(width=400, height=400)
root.geometry("600x500")
Explanation: Running the above code will create an empty top-level Tkinter widget with the title library. And we have set the minimum height and width of the window and geometry.
Add a Background Image to the window
Explanation: We load the image using .open() method and store it in background_image variable. we get the dimension of the image and with the help of resizing function, we resize the image according to the size of the window.
here we have used one dummy variable to increase or decrease the height of an image. If you are using different window geometry then, by increasing or decreasing then, you can fix the image dimension at your window.
- .PhotoImage():- the method id used to display the image(either grayscale or true-color images) in labels, canvas, buttons, or text widgets.
- canvas: canvas is used to add structured graphics to the python application. here we have created an image on canvas using the create_image() method and passing width, height, and image to it as a parameter. and configure it with the new width and height of an image. we use the .pack() method to organize widgets in a block, before placing them into the main parent widget(root).
Setting the Heading Frame
we have added a frame to the parent widget which contains a label of heading. Label() widget is used to add labels and we have added some designing parameters to it like background color, font color, and font. the place() method is used to add a particular widget to the main window at a particular height and width with flexible margin space in x and y-direction.
Adding a Buttons
2) AddBook.py
Implement a function to add a new book to the library
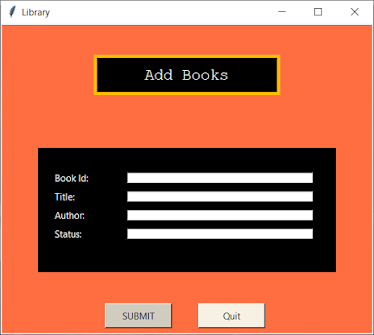
2.1. Import Modules
Import all the necessary modules.
from tkinter import *
from PIL import Image, ImageTk
from tkinter import messagebox
import pymysql
2.2. Function BookRegister()
First, we fetch the data with help of getting () method from the form. after getting the data we are ready to execute the SQL command and we execute this under the try-except block to handle the run time exception efficiently. If entered details are right and the connection is oriented then, your changes will be committed fine to a database.
2.3. Function AddBook()
This function connects to a database(MySQL) and creates a window containing a text field where the user can enter book details and buttons to submit and quit for adding a new book. after clicking the submit button it will call the bookRegister() function, which will fetch the entered data and commit the changes.
We have used a different variable to store each item and one is for connection and cursor object.
- bookInfo1: It contains book Id
- bookInfo2: It contains the title of a book.
- bookInfo3: contains the book Author.
- bookInfo4: contains the status of the book(available, issued, not available).
- con: MySQL console connection object
- cur: Cursor object to the console
- Submit: to submit the changes
- Exit: to quit the window.
We declare certain variables global to use in the bookRegister() function as well. We create another window for AddBooks same as main.py only designing is different, the structure is the same. we pass the cursor control over the shell(cur). It means whatever we want to perform on MySQL shell, will do it through cur. To commit the changes, we will use the connection object(con).
- 📌 we draw a canvas and design it with a background color. we have added a headingLabel inside the headingFrame and given it a title as "Add Books".
- 📌 we create the labelFrame, which basically creates the black box to display the input files to enter book details.
- 📌 In this LabeFrame, we create labels like book Id, title, author, and place them using the place() method with appropriate dimensions. In corresponding to each Label we provide an input text field using Entry().
- 📌 Finally, at end of the window, we add a button to submit the details to add book and exit in case a user does not intend to add the details.
3) ViewBooks.py
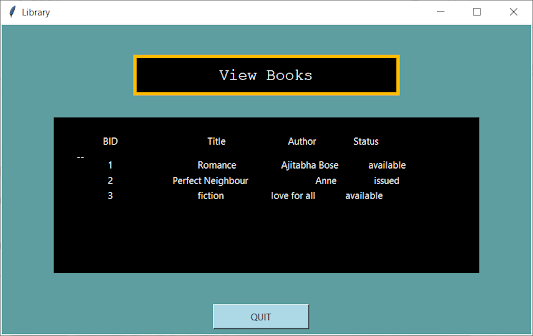
3.1) Import the necessary modules
3.2) Connect to MySQL Server
write the same code to connect to the MySQL server as we have written in the main.py file.3.3) Function - View()
Explanation:
- 👉 First, we create a new window to display the list of books and their status.
- 👉 just like we did in the previous file, we create a headingFrame and a title label to it as "View Books" and same as previous we again create a black box to display the list of books by executing a simple query under the getBooks command.
- 👉 we manually display the name of columns associated with our book table. we execute the query using the .execute() method and we run a for loop to display one by one row with the y-axis difference of 0.1.
- 👉 To handle any exception, we place the execution code in the try-except block.
- 👉 In the end, we also add a quit button to the view books window to close after having a look at the list of books.
4) DeleteBooks.py
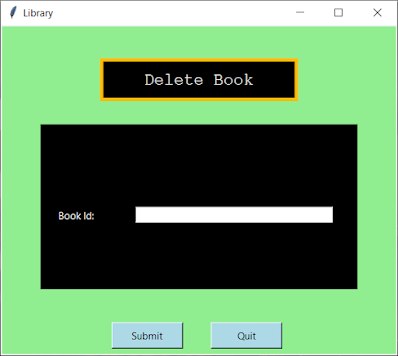
4.1. Import all the necessary modules
4.2. Connect to MySQL database
4.3. Function - DeleteBook()
4.4 Function - delete()
5) IssueBook.py
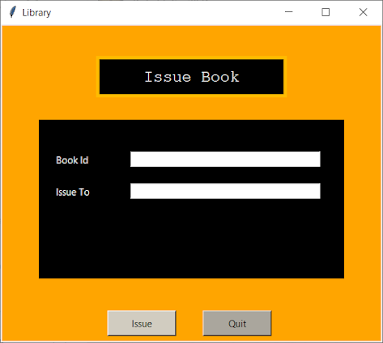
5.2. Connect to MySQL server
5.3. Function - issue()
5.4. Function - issueBook()
6) ReturnBook.py
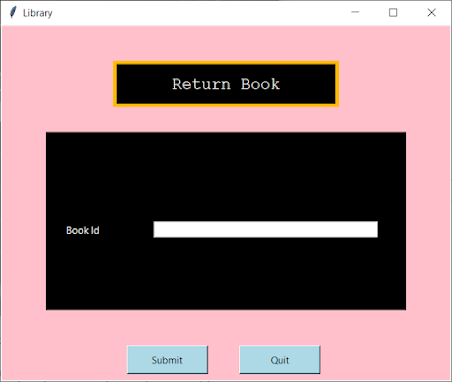
6.1. Import Modules
6.2. Connect to MySQL server
6.3. Function - Return()
The function is very similar to issue() function we designed for issueBook() function.
In this function, we fetch the desired book Id and store it in a bid which book is to be returned. Then, we retrieve all the bids from the books table by executing the extracted command. After that we check the existence of bid in allbid, also we check the status of a particular book is "issued", and if we get status as True then the book is returned successfully by changing the status as "available" and showing a success message to a user.
6.4. Function - ReturnBook()
we create and place a heading frame with the title label of the window. After that, we take the book Id as input. Then, we create two buttons to submit or quite a task to make your submission successful.
Summary
Hooray! We have successfully designed a complete Library Management system using python with a decent UI which is working fine. The project can look a little bit hazy during implementation due to the large codebase, but it's straightforward to understand and implement, and I hope that you are also capable of catching the concepts used very easily.
👉 In this project, we have divided the various task into different files in a particular folder. In real-world scenarios, the same practice is followed to make things easy to understand and build. And constructing any package or library follows the same process.
👉 Apart from this, you can extend this project to an advanced level by implementing a history tab, which will keep the track of all the daily library activities, how many books and which books are been issued by whom, and when. Moreover, you can also integrate a login system to authenticate a user before making any changes to a database.
👉 If you are having any queries then, please post them out in the comment section below 👇, I will very much happy to help you out and it can help someone to learn something new.
Awesome
ReplyDeleteToo good
ReplyDeleteAmazing project.
ReplyDeleteThank you for complete explanation.
Very nice for beginners
ReplyDeleteThanks for in-depth explanation
How to install this project after compete programming
ReplyDeleteI have a question that if there are a lot of books in our database then how we can see all of them as in mine the window cuts off when i try to see more
ReplyDeletePlease Help